Mastering Static Site Generation with Next.js: Your Ultimate Guide
Discover the power of static site generation with Next.js in this comprehensive guide. Learn how to build fast, scalable websites with improved performance and SEO. From setup to advanced techniques, master the essentials of Next.js for static sites today!

In the ever-evolving world of web development, static site generation (SSG) has emerged as a powerful approach for building fast, SEO-friendly, and reliable websites. Among the various tools available for SSG, Next.js stands out due to its robust feature set and seamless integration with modern web technologies. This guide delves into the core concepts of static site generation with Next.js, exploring its benefits, implementation strategies, and best practices.
What is Static Site Generation?
Static Site Generation (SSG) refers to the process of pre-rendering HTML pages at build time. Unlike traditional server-side rendering (SSR) where pages are generated on-the-fly for each request, SSG creates static HTML files during the build process, which are then served to users. This results in faster load times and improved performance, as the server does not need to dynamically generate pages for each request.
Why Choose Next.js for Static Site Generation?
Next.js, a popular React framework, has gained significant traction for its exceptional static site generation capabilities. Here’s why Next.js is a preferred choice:
Optimized Performance
Next.js offers built-in optimizations that enhance performance. The framework supports automatic static optimization, which means pages are pre-rendered and served as static files when possible. This reduces server load and delivers faster response times.
Incremental Static Regeneration (ISR)
One of Next.js's standout features is Incremental Static Regeneration (ISR). ISR allows you to update static content incrementally without needing to rebuild the entire site. This is particularly useful for sites with frequently changing content, ensuring that updates are reflected without compromising performance.
Seamless Integration with React
Next.js leverages React, a powerful JavaScript library for building user interfaces. This integration enables developers to build dynamic and interactive components while still benefiting from static site generation.
Comprehensive Routing
Next.js offers a file-based routing system that simplifies the creation of pages and routes. Each file in the pages
directory corresponds to a route, making it easy to manage and structure your application.
Enhanced SEO Capabilities
Static sites are inherently more SEO-friendly due to their fast load times and pre-rendered content. Next.js further enhances SEO by allowing developers to customize meta tags, manage dynamic content, and integrate with various SEO tools.
Getting Started with Next.js for Static Site Generation
To get started with static site generation using Next.js, follow these steps:
Setting Up Your Development Environment
Before diving into development, ensure you have Node.js and npm (Node Package Manager) installed on your machine. You can download and install them from the official Node.js website.
Creating a New Next.js Project
To create a new Next.js project, open your terminal and run the following command:
npx create-next-app@latest my-nextjs-site
This command initializes a new Next.js project in a directory named my-nextjs-site
. You can replace my-nextjs-site
with your preferred project name.
Exploring the Project Structure
Once the project is created, navigate to the project directory:
cd my-nextjs-site
The basic project structure includes the following directories and files:
pages/
- Contains your application's routes and components.public/
- Stores static assets like images and fonts.styles/
- Includes CSS files for styling your application.next.config.js
- Configuration file for Next.js settings.
Creating Static Pages
To create static pages, add files to the pages
directory. For example, to create a home page, create a file named index.js
:
// pages/index.js import Head from 'next/head'; export default function Home() { return ( <div> <Head> <title>My Next.js Site</title> <meta name="description" content="A static site generated with Next.js" /> </Head> <h1>Welcome to My Next.js Site</h1> <p>This is a static site generated using Next.js.</p> </div> ); }
Adding Dynamic Content with getStaticProps
Next.js allows you to fetch data at build time using the getStaticProps
function. This is useful for displaying dynamic content on static pages. Here’s an example:
// pages/posts.js import Head from 'next/head'; export async function getStaticProps() { // Fetch data from an API or database const res = await fetch('https://api.example.com/posts'); const posts = await res.json(); return { props: { posts, }, }; } export default function Posts({ posts }) { return ( <div> <Head> <title>Posts</title> <meta name="description" content="A list of posts" /> </Head> <h1>Posts</h1> <ul> {posts.map((post) => ( <li key={post.id}>{post.title}</li> ))} </ul> </div> ); }
Implementing Incremental Static Regeneration (ISR)
To enable ISR, you can add a revalidate
key to the object returned by getStaticProps
. This key specifies the number of seconds after which a page re-generation should occur:
export async function getStaticProps() { // Fetch data from an API or database const res = await fetch('https://api.example.com/posts'); const posts = await res.json(); return { props: { posts, }, revalidate: 60, // Revalidate every 60 seconds }; }
Deploying Your Next.js Site
Next.js applications can be deployed to various platforms, including Vercel, Netlify, and traditional hosting providers. To deploy on Vercel, follow these steps:
-
Install Vercel CLI:
npm install -g vercel
-
Deploy Your Site:
vercel
Follow the prompts to deploy your site. Vercel automatically detects your Next.js project and handles the deployment process.
Best Practices for Static Site Generation with Next.js
To maximize the benefits of static site generation with Next.js, consider the following best practices:
Optimize Images and Assets
Optimize images and other static assets to reduce load times and improve performance. Use tools like ImageOptim or Squoosh to compress images without losing quality.
Leverage Caching
Utilize caching strategies to further enhance performance. Implement HTTP caching headers and leverage Content Delivery Networks (CDNs) to distribute content efficiently.
Utilize Next.js Features
Take advantage of Next.js features such as getStaticPaths
for dynamic routes and next/image
for optimized image handling. These features streamline development and enhance the performance of your site.
Monitor Performance and SEO
Regularly monitor your site’s performance and SEO metrics using tools like Google Lighthouse and Google Search Console. Make necessary adjustments to ensure optimal performance and search engine visibility.
Keep Dependencies Up-to-Date
Regularly update your project dependencies, including Next.js, to benefit from the latest features, improvements, and security patches.
Static site generation with Next.js offers a powerful approach for building fast, reliable, and SEO-friendly websites. By leveraging Next.js's features such as Incremental Static Regeneration, automatic static optimization, and seamless integration with React, developers can create high-performing static sites with ease.
Whether you’re building a personal blog, a corporate website, or an e-commerce platform, Next.js provides the tools and flexibility needed to deliver a superior user experience. Embrace the power of static site generation with Next.js and unlock new levels of performance and efficiency for your web projects.
Frequently Asked Questions (FAQs)
What is the MDN HTTP Observatory?
The MDN HTTP Observatory is a tool developed by Mozilla to help web developers identify and rectify security vulnerabilities in their websites, particularly related to HTTP headers and security configurations.
Why was the HTTP Observatory moved to MDN?
Mozilla moved the HTTP Observatory to MDN Web Docs to integrate it with a leading educational resource for developers. This move aims to provide better documentation, updated functionality, and an improved user experience for developers learning about web security.
What types of security tests does the MDN HTTP Observatory perform?
The tool tests for a wide range of security headers and configurations, including Content Security Policy (CSP), Secure Cookies, Cross-Origin Resource Sharing (CORS), HSTS, X-Content-Type-Options, Referrer Policy, Frame Ancestors, and Subresource Integrity (SRI), among others.
How does the Observatory grade websites?
The MDN HTTP Observatory assigns a score and grade (from F to A+) based on how well the website adheres to security best practices. The goal is to encourage developers to aim for an A+ rating by improving their security configurations.
Is achieving an A+ grade enough to ensure my site is fully secure?
Not necessarily. While an A+ grade reflects strong adherence to security best practices, the Observatory doesn't cover all potential vulnerabilities, such as software versions or SQL injections. It’s essential to use the Observatory alongside other security tools for comprehensive protection.
Can the HTTP Observatory test API endpoints?
Yes, but it is primarily designed for websites. It can be used to test API endpoints, though the results may not fully reflect the security status of the API. Using HTTPS and securing headers for APIs is still recommended.
How can I improve my security score?
You can improve your score by following the recommendations provided by the Observatory after each scan. This may include adding or updating security headers, enforcing HTTPS, setting up a Content Security Policy, and more.
What’s new after the migration to MDN?
The migration to MDN brought a more user-friendly interface, modernized tests that reflect the latest security practices, and improved documentation. Outdated tests were removed, and new ones, such as Cross-Origin-Resource-Policy (CORP), were added.
Is the MDN HTTP Observatory free to use?
Yes, the MDN HTTP Observatory is a free tool provided by Mozilla. Developers can use it to scan and improve their websites' security without any cost.
Can the MDN HTTP Observatory help prevent specific attacks?
Yes, by correctly configuring security headers, the tool helps protect against common attacks like Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), Manipulator-in-the-Middle (MiTM) attacks, and others.
Get In Touch
Website – https://www.webinfomatrix.com
Mobile - +91 9212306116
Whatsapp – https://call.whatsapp.com/voice/9rqVJyqSNMhpdFkKPZGYKj
Skype – shalabh.mishra
Telegram – shalabhmishra
Email - info@webinfomatrix.com
What's Your Reaction?


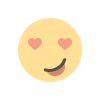
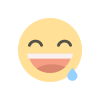


